ReactJs.NET
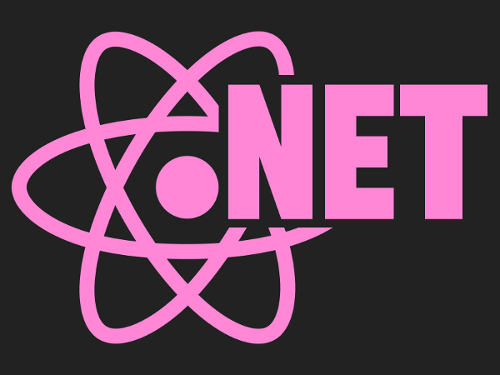
REACTJS
React is an open-source JavaScript library that is used for building user interface. It gives excellent response speed when user inputting using the new method (JSX) to render web page. React allows developers to create large web applications that can change data, without reloading the page. The main purpose of React is to be fast, scalable, and simple. However, React works only on user interfaces in the application. React corresponds to the view in the MVC template. In addition to it can be used with another JavaScript libraries or frameworks such as : Angular JS in MVC.
JSX
In React, in addition to using regular JavaScript for templating, it uses JSX to be faster. JSX is a language that allows to write HTML code in JavaScript. Contrary to JavaScript, JSX is statically-typed, meaning it is compiled before running, just like Java, C ++. So errors are detected right away during compilation.
Why use ReactJs ?
- Simplicity : React uses a special syntax called JSX which allows you to mix HTML with JavaScript.
- Easy to learn : Anyone with a basic previous knowledge in programming can easily understand React .To react, you just need basic knowledge of CSS , JS and HTML.
- Performance with Virtual DOM : When you render a JSX element, every single virtual DOM object gets updated.
REACTJS.NET
ReactJS.NET makes it easier to use React for C# and other.NET languages, especially for ASP.NET MVC ( although it also works with in other environments). It supports both ASP.NET 4 ( with MVC 4 or 5 ), and ASP.NET Core MVC. In addition to, ReactJS.NET is cross-platform so it can run on Linux via Mono or .NET Core.
GETTING STARTED A REACTJS.NET APPLICATION WITH ASP.NET CORE MVC
Start by creating a new ASP.NET Core MVC project
Install packages
Go to the Nuget Package Manager by right-click on the project in the Solution Explorer and select “Manage NuGet Packages…”.
Click the “Browse” tab then search and install packages :
- JavaScriptEngineSwitcher.V8
- React.AspNet
- JavaScriptEngineSwitcher.V8.Native.win-x64
- JavaScriptEngineSwitcher.Extensions.MsDependencyInjection
Set up config
In file Startup.cs
On the top of this file add the code below
using Microsoft.AspNetCore.Http; using JavaScriptEngineSwitcher.V8; using JavaScriptEngineSwitcher.Extensions.MsDependencyInjection; using React.AspNet;
Replace function ConfigureServices with the code below
// This method gets called by the runtime. Use this method to add services to the container. public void ConfigureServices(IServiceCollection services) { services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>(); services.AddReact(); // Make sure a JS engine is registered, or you will get an error! services.AddJsEngineSwitcher(options => options.DefaultEngineName =V8JsEngine.EngineName) .AddV8(); services.AddControllersWithViews(); }
Replace function Configure with the code below
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline. public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Home/Error"); // The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts. app.UseHsts(); } app.UseHttpsRedirection(); app.UseReact(config => { }); app.UseStaticFiles(); app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints = { endpoints.MapControllerRoute( name: "default", pattern: "{controller=Home}/{action=Index}/{id?}"); }); }
Add this to the top of Views\_ViewImports.cshtml
@using React.AspNet
Features
Component
- In React, components act like functions that return HTML components.
- Components are independent and reusable components.
- There are two types of components : Function Component and Class Component.
- Components do the same jobs as functions in JavaScript, but they are independent and return HTML via render function.
Create first component
Create file App.jsx
Create file App.jsx with the code below
class FisrtComponent extends React.Component { render() { return ( <div className="component"> Hello, world! This is a component. </div> ); } } ReactDOM.render(<FisrtComponent />, document.getElementById('content')
Replace the code of the file index.cshtml with the code below
@{ Layout = null; } <html> <head> <title>Hello React</title> </head> <body> <div id="content"></div> <script src="https://cdnjs.cloudflare.com/ajax/libs/react/16.13.0/umd/react.development.js"> </script> <script src="https://cdnjs.cloudflare.com/ajax/libs/react-dom/16.13.0/umd/react-dom.development.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/remarkable/1.7.1/remarkable.min.js"></script> <script src="@Url.Content("~/js/App.jsx")"></script> </body> </html>
At Function Configure in startup.config add the code shown in bold below
app.UseReact(config => { config .AddScript("~/js/App.jsx"); });
Run project
Props
- Props are used to send data to component.
- In React, Props are arguments passed into React components.
- Props are passed to components via HTML attributes.
- Props are constant.
Using props
Use props by using this.props to get value of props as the code below
class FisrtComponent extends React.Component { render() { return ( <div className="Component"> <h1> Hello, world! This is a component. </h1> <h2> This is data from props : {this.props.value}</h2> </div> ); } } ReactDOM.render(<FisrtComponent value = "Props test" />, document.getElementById('content'))
Result
State
- States same as local variables in a tree component and child components can use the state of the parent component.
- States can make change.
- When the state object changes, the component re-renders.
Using State
Create properties of state
constructor(props) { super(props); this.state = { brand: "Ford", color: "Blue", year: 1994 }; }
Use this.setState to set value for this state
this.setState({ color:"Red" })
Create function onclick to change state
changeColor = () => { this.setState({ color:"Red" }) }
Add event to button
<button type="button" onClick={this.changeColor} >Change Color</button>
Result
Before clicked
After clicked
More features
- Hook
- Webpack
- ES6