NODEJS | WRITE LOG WITH WINSTON LIBRARY
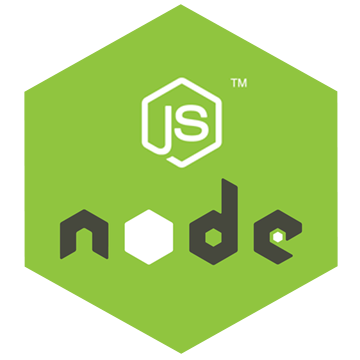
I. INTRODUCTION.
What is winston?
Winston is designed to be a simple and universal logging library with support for multiple transports. Each winston logger can have multiple transports configured at different levels. All logs output to the console or a local file.
Winston aims to decouple parts of the logging process to make it more flexible and extensible.
Installation.
npm install winston or yarn add winston
Include into project.
const winston = require(‘winston’) or import * as winston from ‘winston’.
What’s news (ver 3.2.1)?
Breaking changes.
- Top-level winston.* API.
- Transports
- Container and winston.loggers.
- Logger.
- Exceptions & exception handling.
- Other minor breaking changes.
Upgrading to winston.format.
- Removed winston.Logger formatting options.
- Removed winston.transports.{File,Console,Http} formatting options.
- Migrating filters and rewriters to formats in winston@3.
- Modularity: winston-transport, logform and more.
More detail.
https://github.com/winstonjs/winston/blob/HEAD/UPGRADE-3.0.md
The recommended way to use winston is to create your own logger. The simplest way to do this is using winston.createLogger.
II. LOGGING.
Logging levels in winston conform to the severity ordering specified by RFC5424: severity of all levels is assumed to be numerically ascending from most important to least important.
Parameters.
Name | Default | Description |
level | ‘info’ | Log only if info.level less than or equal to this level |
levels | winston.config.npm.levels | Levels (and colors) representing log priorities |
format | winston.format.json | Formatting for info messages |
transports | [] (No transports) | Set of logging targets for info messages |
exitOnError | true | If false, handled exceptions will not cause process.exit |
silent | false | If true, all logs are suppressed |
The levels provided to createLogger will be defined as convenience methods on the logger returned.
You can add or remove transports from the logger once it has been provided to you from winston.createLogger:
Additional properties for format.
Property | Format added by | Description |
splat | splat() | String interpolation splat for %d %s-style messages. |
timestamp | timestamp() | timestamp the message was received. |
label | label() | Custom label associated with each message. |
ms | ms() | Number of milliseconds since the previous log message. |
As a consumer you may add whatever properties you wish – internal state is maintained by Symbol properties:
Symbol.for(‘level’) (READ-ONLY): equal to level property. Is treated as immutable by all code.
Symbol.for(‘message’): complete string message set by “finalizing formats”:
- json
- logstash
- printf
- prettyPrint
- simple
Symbol.for(‘splat’): additional string interpolation arguments. Used exclusively by splat() format.
NOTE: any { message } property in a meta object provided will automatically be concatenated to any msg already provided: For example the below will concatenate ‘world’ onto ‘hello’:
III. FORMATS.
Formats in winston can be accessed from winston.format. They are implemented in logform, a separate module from winston. This allows flexibility when writing your own transports in case you wish to include a default format with your transport.
If you want to bespoke format your logs, winston.format.printf is for you:
Combining formats.
Any number of formats may be combined into a single format using format.combine.
String interpolation.
The log method provides the string interpolation using util.format. It must be enabled using format.splat().
IV. LOGGING LEVELS.
Each level is given a specific integer priority. The higher the priority the more important the message is considered to be, and the lower the corresponding integer priority. For example, as specified exactly in RFC5424 the syslog levels are prioritized from 0 to 7 (highest to lowest).
Similarly, npm logging levels are prioritized from 0 to 5 (highest to lowest):
Using logging levels
Using custom logging levels.
V. TRANSPORTS.
It is possible to use multiple transports of the same type e.g. winston.transports.File when you construct the transport.
If you later want to remove one of these transports you can do so by using the transport itself.
VI. EXEPTIONS.
Handling Uncaught Exceptions with winston
With winston, it is possible to catch and log uncaughtException events from your process. With your own logger instance you can enable this behavior when it’s created or later on in your applications lifecycle:
If you want to use this feature with the default logger, simply call .exceptions.handle() with a transport instance.
To Exit or Not to Exit.
By default, winston will exit after logging an uncaughtException. If this is not the behavior you want, set exitOnError = false
When working with custom logger instances, you can pass in separate transports to the exceptionHandlers property or set handleExceptions on any transport.
Example 1
Example 2
VII. QUERY LOGS.
Winston supports querying of logs with Loggly-like options.Specifically: File, Couchdb, Redis, Loggly, Nssocket, and Http.
VIII. APPLY INTO PROJECT.
Install winston.
Create winston.ts file.
Write log.
Test.
Using PostMan to excecute api with link http://localhost:8081/v1/user/1 (Id exists).
Using PostMan to excecute api with link http://localhost:8081/v1/user/2 (Id not exists).