Mock data with FakerJs
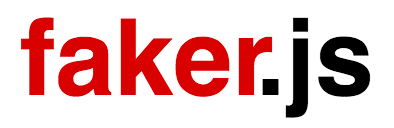
What is FakerJs ?
FakerJs is a JavaScript library that helps generate random data according to different types of data such as email, phone number, address, password, image and so on…
Demo: https://rawgit.com/Marak/faker.js/master/examples/browser/index.html
When is it useful?
- When working with the Front End, we need the data to be processed on the user interface when the real data from the API is incomplete.
- Creates dummy data sent from API when components connecting to database have problems (database, service, host …)
- Generate json sample data for API testing.
NodeJS usage
Via npm:
npm i faker
Import (TypeScript project):
import faker = require("faker");
OR JavaScript project:
var faker = require("faker");
For example, create 3 random Employee objects by FakerJs:
const generateEmployee = () => { return { id: faker.random.uuid(), first_name: faker.name.firstName(), last_name: faker.name.lastName(), email: faker.internet.email(), }; }; //Return 3 object users =>> Array.from({ length: 3 }, generateEmployee)
The result:
[ { "id": "653551ca-29dc-458d-90dd-1c7a04004350", "first_name": "Yadira", "last_name": "Zieme", "email": "Russel_Will88@yahoo.com" }, { "id": "14243402-a6f9-4005-bc61-bb1d25f23183", "first_name": "Nyah", "last_name": "Hermiston", "email": "Sigrid77@gmail.com" }, { "id": "60dbec10-2898-4571-8187-31072f849ed9", "first_name": "Reed", "last_name": "Towne", "email": "Alejandrin.Simonis@hotmail.com" } ]
Faker Js now supports multi language generation. Example:
Define the locale before creating the employee object:
faker.locale = 'ja';
The result:
[ { "id": "c0a9b596-ade2-4d9e-bddc-3a6e6ed43fcb", "first_name": "結菜", "last_name": "加藤", "email": "海翔_井上@gmail.com" }, { "id": "12381746-bb9e-479c-adf0-ba7887392a06", "first_name": "杏", "last_name": "渡辺", "email": "結菜_清水11@gmail.com" }, { "id": "264a8702-3318-43d1-9518-748046ff6ac5", "first_name": "心愛", "last_name": "清水", "email": "結衣_山田57@gmail.com" } ]
Available data types and languages refer to: http://marak.github.io/faker.js/
Seed in FakerJs
FakerJs helps us to generate random data. But in some cases, we need the data generated to be the same in different processes. Seed helps us to solve this:
faker.seed(1); var number1st = faker.random.number(); // name1st = 123 // Setting the seed again resets the sequence. faker.seed(1); var number2nd = faker.random.number(); // number2nd = 123 // => number1st === number2nd
Other services of FakerJs
FakerJs API
The API will return a single data type field according to the parameter when requested
http://faker.hook.io/?property=internet.email&locale=en
The result:
"Ted37@hotmail.com"
Faker Cloud
Quickly create one or more dummy data, very useful for when we need a lot of data to test the API, the command to insert into the database series, etc… Support many formats CSV, JSON, MySQL, MSSQL etc…
Summary:
FakerJs is a handy and compact library. It is suitable for both back-end, front-end and especially FakerCloud which is quite suitable for testers. It simplifies many things that are almost time-consuming to do such as inserting a series of records into the database, create JSON lists to send to the API, support multilingual data, etc.
References: