How to Add Authentication to a Vue.js App Using AWS Amplify
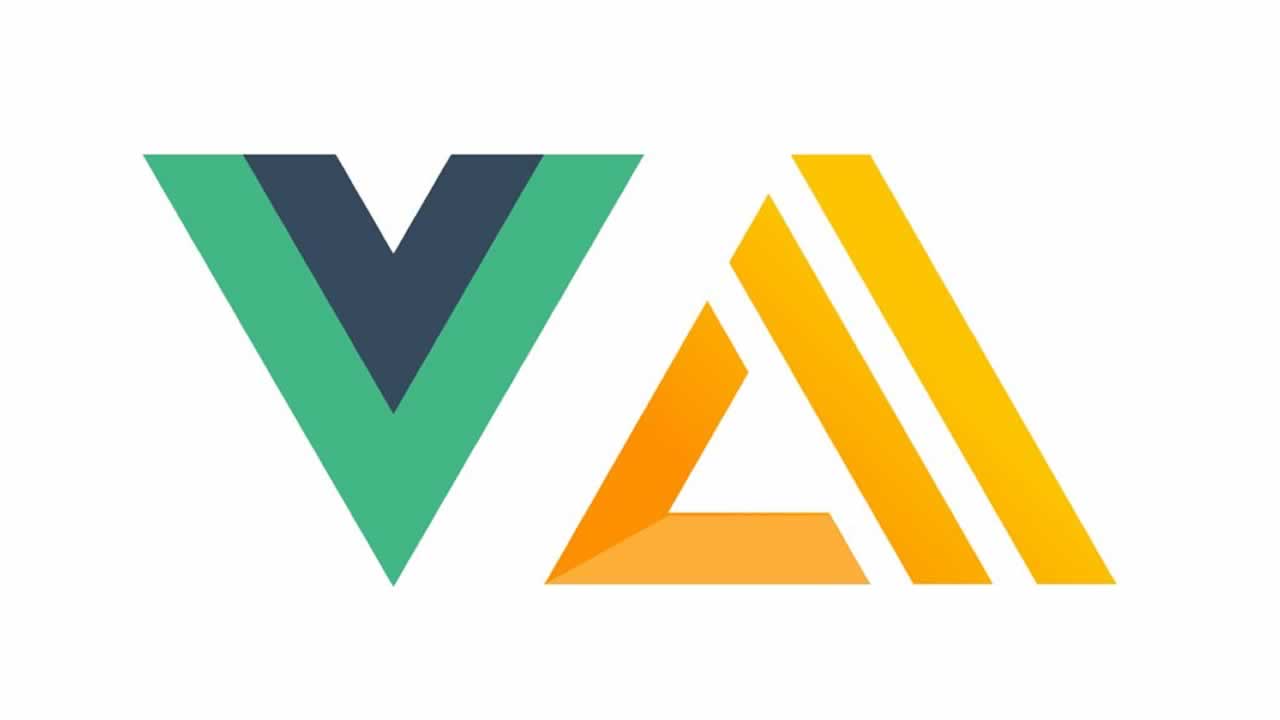
What’s AWS Amplify?
AWS Amplify is an open-source framework created by Amazon that contains a set of tools and services that can be used together or on their own. One of the tools is Amplify Auth. Amplify Auth lets you quickly set up secure authentication and control what users have access to in your application.
The Amplify framework uses Amazon Cognito as the main authentication provider. Amazon Cognito is a robust user-directory service that handles user registration, authentication, account recovery, and other operations.
Create an AWS Account
To get started, you’ll need to create an AWS account here. If you don’t have an AWS account, follow the directions here to create one.
Creating Project
We’ll be using the Vue CLI to scaffold out a project for us to start with. To do that, you need to have the Vue CLI installed on your system. If you don’t have it installed, you can install it with this command:
npm install -g @vue/cli
Now we can use the Vue CLI to create our project. Create a new project using this command:
vue create vue-amplify-auth-tutorial
You’ll be asked to pick a preset. Choose “Manually select features,” and then select “babel,” “Router,” and “Linter / Formatter.”
After the Vue CLI is finished, it’ll give you the commands to change into the new directory that was just created and the command to start the server. Follow those directions. Once the server is started you can open your browser to localhost:8080
.
Install and Configure the Amplify CLI
The Amplify CLI is a unified toolchain to create AWS cloud services for your app. You can install it with this command:
npm install -g @aws-amplify/cli
Next, we need to configure Amplify by running the following command:
amplify configure
This command will open up a new browser window and ask you to sign into the AWS console. Once you’re signed in, return to your terminal, and press Enter.
You’ll be asked to specify the AWS Region. Select the region closest to you.
You’ll need to specify the username of the new IAM user. It’ll provide a default name you can use by hitting enter or you can specify your own name. I’m going to call my user: auth-demo
When you press Enter
, you’ll be taken back to your browser.
Click the Next: Permissions button.
Click the Next: Tags button.
Click the Next: Review button.
Click the Create User button.
Now go back to your terminal and press Enter
to continue.
Type in the accessKeyId
of the user you just created, and press Enter
.
Type in the secretAcessKey
of the user you just created, and press Enter
.
You’ll be asked to enter a profile name. I’ll accept the supplied value (the default) by just pressing Enter
.
When everything is finished, you should get a message in your terminal that the new user was successfully set up.
Create the Authentication Service
From the root of your Vue application, run:
amplify init
We need to add an authentication service to our Vue application. In the root directory of your Vue application, enter this command:
amplify add auth
When you initialize Amplify, you’ll be prompted for some information about your application.
Enter a project name:
Set the back-end environment name to be:
Select your preferred code-editor software:
We’re using Vue, so select that as our JavaScript framework.
This is the default, so you can just press Enter
to continue.
When you initialize a new Amplify project, a few things happen:
- It creates a top-level directory called
amplify
that stores your back-end definition. - It creates a file called
aws-exports.js
in thesrc
the directory that holds all of the configurations for the services you create with Amplify. This is how the Amplify client is able to get the necessary information about your back-end services.
To deploy the service, run the amplify
push
command.
Install Amplify Libraries
We need to install the Amplify dependencies in our Vue application. You can install them with this command:
npm install aws-amplify
Configure Application
We need to add Amplify to our Vue application. Open up the main.js
file, and add the following after the last import line.
import Amplify from 'aws-amplify'; import awsconfig from './aws-exports'; Amplify.configure(awsconfig);
Create a Sign-Up Page
When a user submits the form it calls the register
method. Here’s the code for that method:
async register() { try { await Auth.signUp({ username: this.email, password: this.password, }); alert('User successfully registered. Please login'); } catch (error) { alert(error.message); } },
This method uses Auth
from the aws-amplify
package we installed. Add this import for it at the beginning of the script section.
import { Auth } from 'aws-amplify';
Now open up your application, and register a new user. If successful, you’ll get an alert saying the user was registered.
Create a Login Page
We need to implement is the login method. Here’s the code:
async login() { try { await Auth.signIn(this.email, this.password); alert('Successfully logged in'); } catch (error) { alert(error.message); } },
Now if you open up your application, you’ll be able to log in with the user you previously registered.
Adding a Logout Method
In the script section, add a methods
object, and include the logout
method. It should look like this:
methods: { async logout() { try { await Auth.signOut(); } catch (error) { alert(error.message); } }, },
Congratulations, you’ve successfully added AWS Amplify authentication to your Vue application.
Link the code from Gitlab.
References:
https://aws.amazon.com/amplify/
https://blog.thundra.io/how-to-build-an-application-in-minutes-with-aws-amplify